设数据表Users:
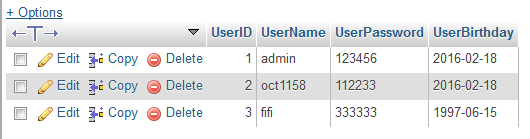
则在PHP中可以定义一个User类和基类DataObject,使得访问数据表可以用下面的方法:
<?php
include_once('conn.php');
include_once('DataObject.php');
include_once('User.php');
// 添加一个新用户
$user = new User();
$user->name = 'oct1158';
$user->password = '789012';
$user->useFunction('birthday', 'NOW()');
echo 'Field birthday uses MySQL Function: ', $user->birthday, '<br>';
if ($user->insert()) {
echo 'New User ID: ', $user->id, '<br>';
// 更新用户信息
$user->password = '112233';
$user->update();
} else {
echo 'INSERT Failed<br>';
}
// 获取一个用户
$sql = 'SELECT * FROM Users WHERE UserName = ?';
$stmt = $dbh->prepare($sql);
$stmt->execute(array('admin'));
$admin_user = $stmt->fetchObject('User');
echo 'Admin ID is ', $admin_user->id, '.<br>';
echo 'Admin Birthday is ', $admin_user->birthday, '.<br>';
$users = User::GetAll();
echo '<br>';
echo $users[0]->name, ', ', $users[0]->birthday, '<br>';
echo $users[1]->name, ', ', $users[1]->birthday, '<br>';
echo $users[2]->name, ', ', $users[2]->birthday, '<br>';
echo '<br>';
// 删除一个用户
$user = new User();
$user->insert();
$user->delete();